JOGLで樽の形を描画するには、以下のコードを実行します。
import java.io.*;
import javax.media.opengl.*;
import com.sun.opengl.util.*;
import com.sun.opengl.util.texture.*;
public class JoglSample31
{
// 出力画像サイズ
private static int width = 300;
private static int height = 300;
public static void main(String args[])
throws IOException
{
GLDrawableFactory gldf =
GLDrawableFactory.getFactory();
GLCapabilities glc = new GLCapabilities();
glc.setDoubleBuffered(false);
GLPbuffer buf = gldf.createGLPbuffer(
glc, null, width, height, null);
buf.addGLEventListener(
new GLEventListener(){
// 初期化
public void init(GLAutoDrawable dr)
{
GL gl = dr.getGL();
// 背景色
gl.glClearColor(
(float)0xf0/(float)0xff,
(float)0xf0/(float)0xff,
(float)0xf0/(float)0xff,
1f);
try
{
Texture texture = TextureIO.newTexture(
new File("sf.jpg"), true);
texture.enable();
texture.bind();
}
catch(IOException ioex){}
}
public void display(GLAutoDrawable dr)
{
GL gl = dr.getGL();
GLUT glut = new GLUT();
gl.glViewport(0, 0, width, height);
// 透視投影
gl.glMatrixMode(GL.GL_PROJECTION);
gl.glLoadIdentity();
float ratio = (float)height/(float)width;
gl.glFrustum(-1.0f, 1.0f, -ratio, ratio,
5.0f, 40.0f);
gl.glMatrixMode(GL.GL_MODELVIEW);
gl.glLoadIdentity();
gl.glTranslatef(0.0f, 0.0f, -10.0f);
gl.glEnable(GL.GL_LIGHTING);
gl.glEnable(GL.GL_LIGHT0);
gl.glEnable(gl.GL_COLOR_MATERIAL);
gl.glEnable(GL.GL_NORMALIZE);
gl.glEnable(GL.GL_DEPTH_TEST);
// gl.glEnable(GL.GL_CULL_FACE);
gl.glClear(GL.GL_COLOR_BUFFER_BIT |GL.GL_DEPTH_BUFFER_BIT );
gl.glPushMatrix();
// 面の色を設定
gl.glColor3f(
(float)0x77/(float)0xff,
(float)0x99/(float)0xff,
(float)0xff/(float)0xff
);
// X軸回転
gl.glRotatef(30.0f, 1.0f, 0.0f, 0.0f);
// Y軸回転
gl.glRotatef(50.0f, 0.0f, 1.0f, 0.0f);
//
double minr = 0.7f;
double maxr = 1.0f;
int slices = 16;
double lr=2*Math.PI/slices;
double dh = 0.5;
double th = 2.5;
double cx[] = new double[slices];
double cy[] = new double[slices];
for(int li=0;li<slices;li++){
double x1 = minr*Math.cos(lr*(li+1));
double y1 = minr*Math.sin(lr*(li+1));
double x2 = maxr*Math.cos(lr*(li+1));
double y2 = maxr*Math.sin(lr*(li+1));
double x3 = maxr*Math.cos(lr*li);
double y3 = maxr*Math.sin(lr*li);
double x4 = minr*Math.cos(lr*li);
double y4 = minr*Math.sin(lr*li);
cx[li] = x4;
cy[li] = y4;
drawQuads(gl,
x4, y4, -th/2,
x3, y3, -th/2+dh,
x2, y2, -th/2+dh,
x1, y1, -th/2);
drawQuads(gl,
x2, y2, -th/2+dh,
x3, y3, -th/2+dh,
x3, y3, th/2-dh,
x2, y2, th/2-dh);
drawQuads(gl,
x1, y1, th/2,
x2, y2, th/2-dh,
x3, y3, th/2-dh,
x4, y4, th/2);
}
gl.glBegin(GL.GL_POLYGON);
gl.glNormal3d(0,0,-1);
for(int li=0;li<slices;li++){
gl.glVertex3d(cx[li], cy[li], -th/2);
}
gl.glEnd();
gl.glBegin(GL.GL_POLYGON);
gl.glNormal3d(0,0,1);
for(int li=0;li<slices;li++){
gl.glVertex3d(cx[li], cy[li], th/2);
}
gl.glEnd();
gl.glPopMatrix();
}
public void reshape(
GLAutoDrawable dr,
int x, int y,
int width, int height){}
public void displayChanged(
GLAutoDrawable dr,
boolean modeChanged,
boolean deviceChanged){}
private void drawQuads(GL gl,
double p1x, double p1y, double p1z,
double p2x, double p2y, double p2z,
double p3x, double p3y, double p3z,
double p4x, double p4y, double p4z)
{
gl.glBegin(GL.GL_QUADS);
gl.glNormal3d(p1x, p1y, p1z);
gl.glVertex3d(p1x, p1y, p1z);
gl.glNormal3d(p2x, p2y, p2z);
gl.glVertex3d(p2x, p2y, p2z);
gl.glNormal3d(p3x, p3y, p3z);
gl.glVertex3d(p3x, p3y, p3z);
gl.glNormal3d(p4x, p4y, p4z);
gl.glVertex3d(p4x, p4y, p4z);
gl.glEnd();
}
}
);
GLContext context = buf.createContext(null);
context.makeCurrent();
buf.display();
Screenshot.writeToFile(
new File("sample1189a.png"), width, height, true);
context.release();
context.destroy();
}
}
出力画像(sample1189a.png)
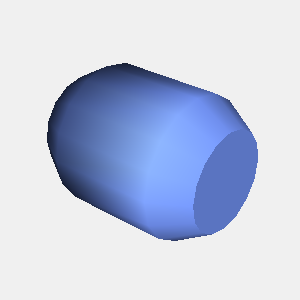
動作環境
JDK6 Upadate13, JOGL 1.1.1